Taking One From The Team
/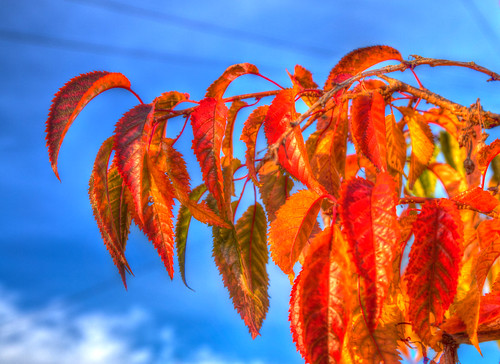
One of the Second Year courses that I help deliver is our Software Development module. As part of that we put our students into teams and get them to write some software for a picky customer. They have to deal with dodgy legacy software and the fun and games that is associated with working as part of a team.
One of the rules that we have is that everyone in a team should write some code. Not all of it, but some of it. One of our worries is that in a team of 6 people we might get a couple of folks who will say “We’re the best programmers here, we should write all the code” and then go ahead and try to do just that. This is bad in many, many ways.
For a start, they might not be the best programmers there, just the ones with the biggest egos/mouths. For another thing, they might not be able to do all the work with just two people. But most importantly, if they are the best programmers around, and they could write all the code they should still not try to do it. Because from a learning outcome point of view they are missing out on a huge opportunity.
If the “great programmers” just churn out the code all they’ve done is reinforce their high opinion of themselves. But if instead they decided to piece out the work sensibly and then spend some of their time mentoring those who are less confident coders so that everyone gets better at development, then they are picking up an incredibly valuable skill. The ability to teach people stuff is really useful, even if you have no intention of going into teaching. When you try to explain something to another person you have to try to put yourself in their position and then find a context which they understand, into which you can put the information you are delivering.
The skill of being able to explain something to another person is very valuable, and it is just the kind of thing that employers are looking for. It also makes you more confident in interview situations, just because you are better at talking to people.
So, don’t think that offering to write all the code is “taking one for the team”. It is more like “taking one from the team”.